Editing EPUB metadata can significantly enhance the usability and organization of digital books. Whether it’s for personal organization, redistributing ebooks, or fixing errors in downloaded files, modifying EPUB metadata can make your digital library more functional and visually appealing. Python, with its simplicity and versatility, provides a powerful option for tackling this task with ease.
This beginner-friendly guide will walk you through the process of editing EPUB metadata using Python, leveraging libraries like ebooklib
. You’ll learn how to unpack EPUB files, modify metadata, and repack the files into their original format—all with minimal coding experience required.
Understanding EPUB Metadata
Metadata is essential for describing the content of any EPUB file. Common metadata elements include:
- Title: The name of the book.
- Author: The creator of the work.
- Publisher: The company or person who published the book.
- Language: The language in which the book is written.
- Identifiers: Unique IDs such as an ISBN.
By modifying metadata, users can correct errors, standardize naming conventions, or personalize their collections. Python provides an efficient way to handle this, especially for managing multiple files.
Getting Started with Python
Follow these steps to begin editing EPUB metadata:
1. Install Required Libraries
First, install the ebooklib
library, which is specifically designed for working with EPUB files. Use the following command:
pip install ebooklib
You may also need lxml
, which can be installed with:
pip install lxml
2. Access EPUB Metadata
To extract and edit metadata, write a basic Python script using ebooklib
. Here’s a simple example:
from ebooklib import epub
# Load the EPUB file
book = epub.read_epub('example.epub')
# Access the metadata
title = book.get_metadata('DC', 'title')
author = book.get_metadata('DC', 'creator')
print('Title:', title)
print('Author:', author)
This script extracts the title and author of the book. The get_metadata()
function allows you to retrieve any metadata field, given its namespace and name.
3. Modify EPUB Metadata
To edit metadata, use the set_metadata()
function:
# Update the title and author
book.set_metadata('DC', 'title', 'New Title')
book.set_metadata('DC', 'creator', 'New Author')
# Save the updated EPUB
epub.write_epub('updated_example.epub', book)
Once you make changes, the updated version of the EPUB file is saved. This process is simple, yet powerful for managing your library effectively.
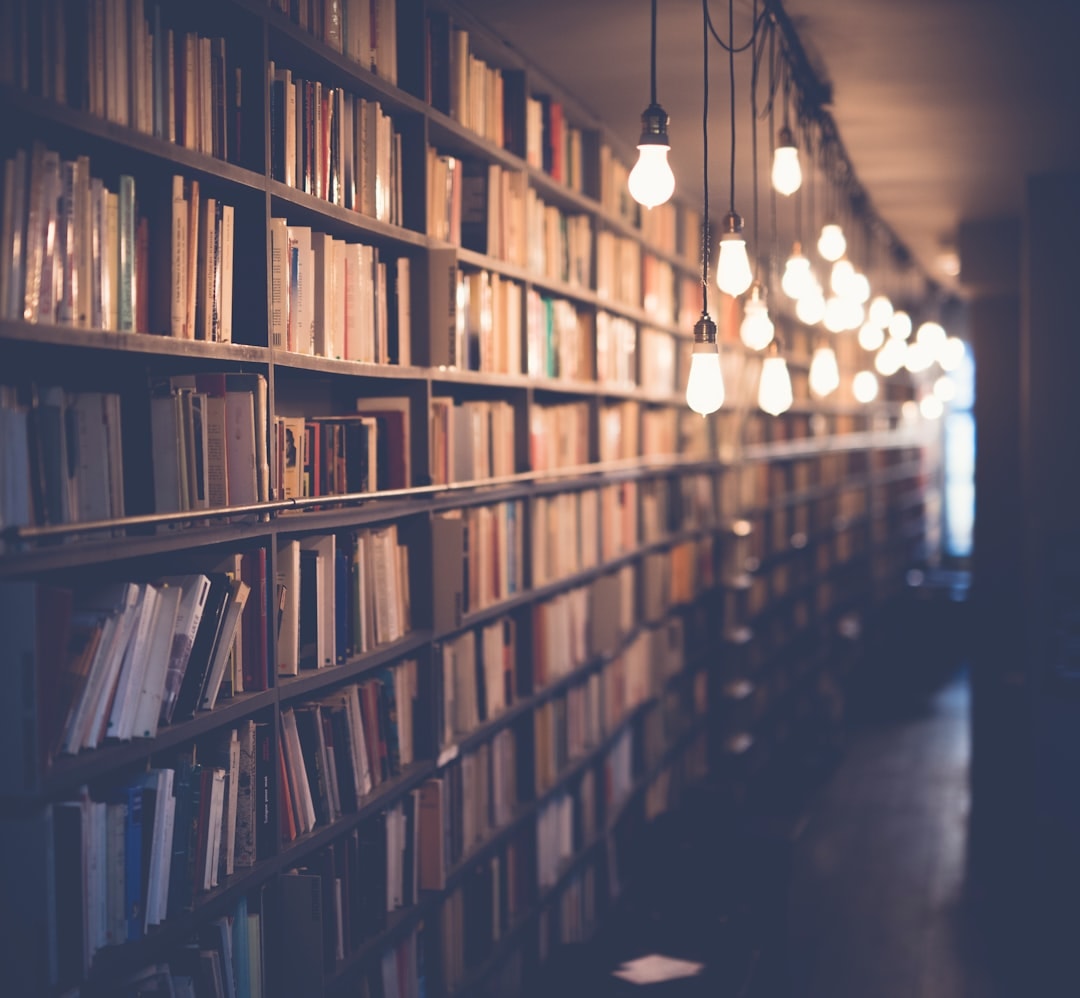
Additional Tips and Best Practices
- Backup Your Files: Always keep a copy of the original EPUB file before making modifications.
- Batch Processing: Consider writing a loop to automate metadata editing for multiple EPUB files in the same directory.
- Understand EPUB Structure: EPUB files are essentially ZIP archives containing XML files. Familiarity with the structure can help troubleshoot errors.
With Python, editing EPUB metadata becomes not only efficient but also an opportunity to gain hands-on experience with programming concepts.
Possible Challenges
While working with EPUB files, you might encounter the following issues:
- Some EPUB files may lack standardized metadata, which could require manual intervention.
- Errors in the XML structure of an EPUB file may result in warnings or failed operations.
In such cases, inspecting the contents of the EPUB file directly (by unzipping it) can help you identify and correct issues.
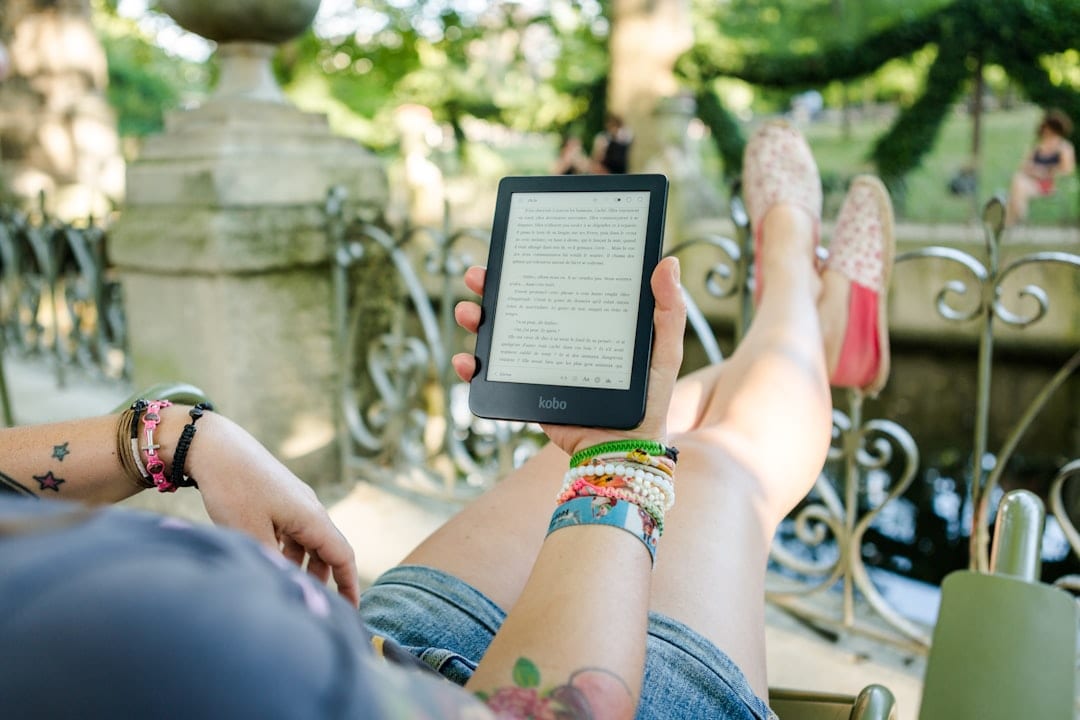
Conclusion
Editing EPUB metadata with Python is a highly efficient way to manage your digital book collection. Using libraries like ebooklib
, even beginners can extract, modify, and repack EPUB files with ease. By mastering these techniques, you can ensure your ebooks are correctly categorized, easily searchable, and visually appealing in e-reader applications.
FAQ
- What is EPUB metadata?
- EPUB metadata refers to information about the book, such as title, author, publisher, language, and identifiers.
- Why should I edit EPUB metadata?
- Editing metadata allows you to fix errors, standardize file naming, and improve ebook organization and searchability.
- Can I edit metadata in bulk?
- Yes, you can extend the Python script to process multiple EPUB files in a folder using loops.
- Are there risks involved in modifying EPUB metadata?
- The main risk is corrupting the file, but this can be avoided by keeping a backup of the original EPUB files before making changes.
- Do I need advanced Python skills for this?
- No, the process is beginner-friendly and requires only basic Python knowledge.